Hls.js
Tuesday, March 31, 2020 5:24 AM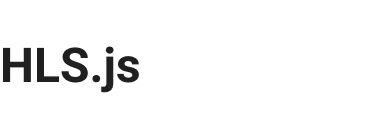
hls.js is a JavaScript library that implements the HTTP Live Streaming client. It uses HTML5 video and MediaSource Extensions for playback.
Supported versions of HLS.js
Teleport.js is compatible with HLS.js version 0.7.0 and later.
Before start
Before teleport.js in initialized the first time, you need to validate the domain and get the API key in Teleport client area.
Initialization
To load chunks from P2P you need:
- Create an instance of HLS.js.
- Initialize Teleport with loader params and API key as an argument.
Example
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>TELEPORT HLSJS EXAMPLE</title>
</head>
<body>
<video id="video" width="640" controls autoplay></video>
<script src="https://cdn.jsdelivr.net/hls.js/latest/hls.min.js"></script>
<script src="https://cdn.teleport.media/stable/teleport.hlsjs.bundle.js"></script>
<script>
var tlprt;
var STREAM_URL = "<manifest URL>";
var API_KEY = "<YOUR APIKEY>";
function initApp() {
// Create player
var hls = new Hls();
var video = document.getElementById('video');
hls.loadSource(STREAM_URL);
hls.attachMedia(video);
hls.on(Hls.Events.MANIFEST_PARSED, function () {
video.play();
});
// Init Teleport
teleport.initialize({
apiKey: API_KEY,
loader: { // Loader configuration
type: "hlsjs", // Plagin ID
params: {
hlsjs: hls // Player instance
}
}
})
.then(function (instance) {
// save teleport instance
tlprt = instance;
console.log("The video has now been loaded!");
// Subscribe to on close window event for free used resources
window.addEventListener("unload", function () {
if (tlprt) {
tlprt.dispose();
tlprt = null;
}
});
})
.catch(onError);
}
function onError(error) {
// Log the error
console.error("Error code", error.code, "object", error);
}
document.addEventListener("DOMContentLoaded", initApp);
</script>
</body>
</html>