Shaka Player
Tuesday, March 31, 2020 5:24 AM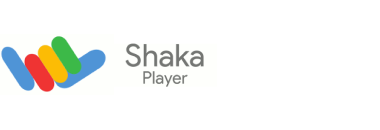
Shaka Player is an open source library for playing adaptive video streams. It supports formats such as HLS and DASH without the use of plug-ins and Flash. Instead, the Shaka Player uses the open Web standards for MediaSource Extensions and Encrypted Media Extensions.
Supported versions of Shaka Player
Teleport.js is compatible with Shaka Player version 2.1.0 or later.
Supported formats
Teleport.js was successfully tested with MPEG Dash. Soon we are expecting HLS.js support.
Required external data
-
apiKey
- before teleport.js in initialized the first time, you need to validate the domain and get the API key in Teleport client area. -
manifest
- the link to .mpd manifest.
Initialization
Loading javascript modules when integrating Shaka Player with teleport.js should occur strictly in the following order:
- Shaka Player.
- Teleport Core bundle with Teleport Shaka Player plugin.
Example:
<script src="https://cdnjs.cloudflare.com/ajax/libs/shaka-player/2.2.6/shaka-player.compiled.js"></script>
<script src="https://cdn.teleport.media/stable/teleport.shaka.bundle.js"></script>
To load chunks from P2P you need:
- Create an instance of Shaka Player.
- Initialize Teleport with loader params and API key as an argument.
Example
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>TELEPORT SHAKA EXAMPLE</title>
<script src="//cdnjs.cloudflare.com/ajax/libs/shaka-player/2.2.5/shaka-player.compiled.debug.js"></script>
</head>
<body>
<video id="video" width="640" controls autoplay></video>
<!-- Include Teleport Core bundle with Teleport Shaka Player plugin -->
<script src="https://cdn.teleport.media/stable/teleport.shaka.bundle.js"></script>
<!-- Setup -->
<script>
var tlprt;
var STREAM_URL = "<manifest URL";
var API_KEY = "<YOUR APIKEY>";
function initApp() {
shaka.polyfill.installAll();
var video = document.getElementById("video");
var player = new shaka.Player(video); // Create player
player.addEventListener("error", onErrorEvent);
// Init teleport
teleport.initialize({
apiKey: API_KEY,
loader: { // Loader configuration
type: "shaka", // Plugin ID
params: {
player: player // Player instance
}
}
})
.then(function (instance) {
// Load a manifest and save teleport instance
tlprt = instance;
return player.load(STREAM_URL);
})
.then(function () {
console.log('The video has now been loaded!');
// Subscribe to on close window event for free used resources
window.addEventListener("unload", function () {
if (tlprt) {
tlprt.dispose();
tlprt = null;
}
});
})
.catch(onError);
}
function onErrorEvent(event) {
onError(event.detail);
}
function onError(error) {
// Log the error
console.error("Error code", error.code, "object", error);
}
document.addEventListener("DOMContentLoaded", initApp);
</script>
</body>
</html>