Video.js HLS
Tuesday, March 31, 2020 5:24 AM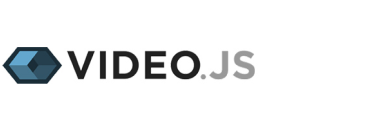
Video.js is an open source library for adaptive video streams playback.
Supported versions of VideoJS
Teleport.js is compatible with VideoJS version 5.19.1 or later.
Before start
Before teleport.js in initialized the first time, you need to validate the domain and get the API key in Teleport client area.
Initialization
Loading javascript modules when integrating Video.js with Teleport.js should occur strictly in the following order:
- Video.js.
- Teleport Core bundle with Teleport VideoJS HLS plugin.
Example:
<script src="https://cdnjs.cloudflare.com/ajax/libs/video.js/6.4.0/video.min.js"></script>
<script src="https://cdn.teleport.media/stable/teleport.videojs-hls.bundle.js"></script>
To load chunks from P2P you need:
- Initialize Teleport with loader params as an argument.
- Create an instance of Video.js.
Important note
If you want to initialize the player with additional parameters, you need to specify them with the second parameter in the player creation function (in the same place as the loader of the Teleport), but not in the data-setup
attribute of the video
tag. Otherwise, the player can be created ahead of time.
Example
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>TELEPORT VIDEOJS HLS EXAMPLE</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/video.js/6.4.0/video-js.min.css">
</head>
<body>
<video id="video" width="600" height="300" class="video-js vjs-default-skin" controls>
<source src="<manifest URL>" type="application/x-mpegURL" />
</video>
<!-- Include VideoJS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/video.js/6.4.0/video.min.js"></script>
<!-- Include Teleport Core bundle with Teleport VideoJS plugin -->
<script src="https://cdn.teleport.media/stable/teleport.videojs-hls.bundle.js"></script>
<!-- Setup -->
<script>
var API_KEY = "<YOUR APIKEY>";
function initApp() {
// Init Teleport
teleport.initialize({
apiKey: API_KEY,
loader: { // Loader configuration
type: "videojs-hls", // Plugin ID
params: {
videojs: window.videojs // Player constructor
}
}
})
.then(function () {
// Create player
var player = window.videojs('video');
player.play();
})
.catch(onError);
}
function onError(error) {
// Log the error
console.error("Error code", error.code, "object", error);
}
document.addEventListener("DOMContentLoaded", initApp);
</script>
</body>
</html>