Clappr HLS
Tuesday, March 31, 2020 5:24 AM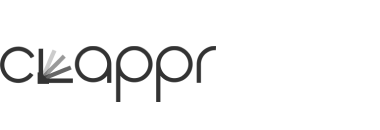
Clappr is an extensible plugin for creating video players that can be used as backgrounds or simple video containers. It is supported by many browsers and basically all mobile devices, even in some video game consoles.
Supported versions of Clappr
Teleport.js is compatible with Clappr versions later than 0.2.70.
Before start
Before teleport.js in initialized the first time, you need to validate the domain and get the API key in Teleport client area.
Initialization
Loading javascript modules when integrating Clappr with teleport.js should occur strictly in the following order:
- Clappr.
- Teleport Core bundle with Clappr HLS plugin.
Example:
<script src="https://cdn.jsdelivr.net/npm/clappr@latest/dist/clappr.min.js"></script>
<script src="https://cdn.teleport.media/stable/teleport.clappr-hls.bundle.js"></script>
To load chunks from P2P you need:
- Initialize Teleport with
clappr-hls
loader. - After initializing the Teleporter script, get the loader API, and then
TeleportClapprHlsPlugin
plugin. It is supports P2P connections. - Create an instance of Clappr with
TeleportClapprHlsPlugin
plugin.
Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>TELEPORT CLAPPR HLS EXAMPLE</title>
</head>
<body>
<div id="video"></div>
<script src="https://cdn.jsdelivr.net/npm/clappr@latest/dist/clappr.min.js"></script>
<script src="https://cdn.teleport.media/stable/teleport.clappr-hls.bundle.js"></script>
<script>
var tlprt;
var STREAM_URL = "<manifest URL>";
var API_KEY = "<YOUR APIKEY>";
function initApp() {
teleport.initialize({
apiKey: API_KEY,
loader: { // Loader configuration
type: "clappr-hls" // Plugin ID
}
})
.then(function (instance) {
tlprt = instance;
// Get plugin for Clappr from loader
var TeleportClapprHlsPlugin = instance.getLoader().getPlugin();
// Create player
var player = new Clappr.Player({
source: STREAM_URL,
autoPlay: true,
plugins: [
TeleportClapprHlsPlugin
],
parentId: "#video"
});
})
.then(function () {
console.log("The video has now been loaded!");
// Subscribe to on close window event for free used resources
window.addEventListener("unload", function () {
if (tlprt) {
tlprt.dispose();
tlprt = null;
}
});
})
.catch(onError);
}
function onError(error) {
// Log the error
console.error("Error code", error.code, "object", error);
}
document.addEventListener("DOMContentLoaded", initApp);
</script>
</body>
</html>